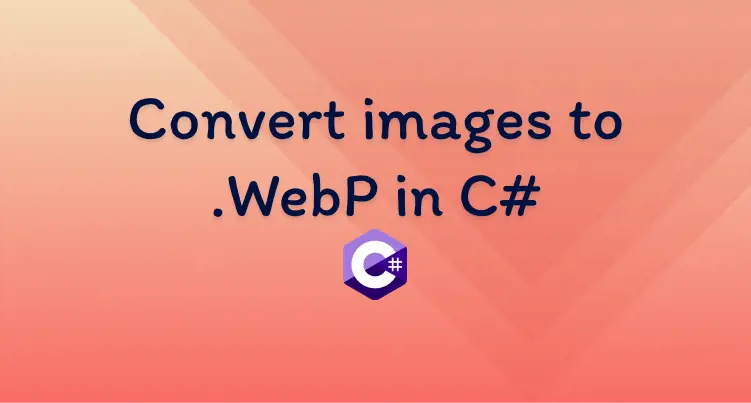
Convert images to WebP in C# .Net 7 (.Net Core)
21 December 2022
·I recently migrated this blog from WordPress to a custom Nuxt site. I moved from WordPress to have more control over the blog and not have to rely on plugins to do everything. It’s worked out really well but there is one plugin I do miss: Smush ☹️
Performance is really important for all websites. It improves user experience and has a big impact on SEO rankings. The standard tool for measuring performance is Google Lighthouse. It’s what I used when I migrated this blog. Lighthouse scores your performance out of 100 and gives suggestions on how to improve it.
Images are often the biggest assets in a blog post and can really damage the Lighthouse score. This is where Smush comes in. Smush does a great job of automatically optimizing your blog’s images. Optimized images helps your blog load quickly and get a good Lighthouse score.
Now that I don’t have access to Smush on my custom Nuxt site I have to optimize the images myself…
An improvement that Lighthouse always suggests is to convert all images to the WebP format. WebP is a replacement for Jpeg, Png and Gif images. It has a much smaller file size which is why Lighthouse suggests it. Converting all my images to it should give the blog a nice boast 🚀
All the images on this blog are served by an Azure function and cached via Cloudflare. This means I can convert them to WebP on the fly inside the function.
Anyway that’s a really long explanation for just a couple lines of code 😅
All you need is the SixLabors.ImageSharp Nuget package and some code like this:
var imageBytes = await File.ReadAllBytesAsync("your-image.jpg");
using var inStream = new MemoryStream(imageBytes);
using var myImage = await Image.LoadAsync(inStream);
using var outStream = new MemoryStream();
await myImage.SaveAsync(outStream, new WebpEncoder());
return new FileContentResult(outStream.ToArray(), "image/webp");
WebP file size example
Lighthouse isn’t lying BTW, the difference in file size is actually really good…
For example, this screenshot of my blog home page goes from 622KB as a PNG to just 72KB as a WebP 👀