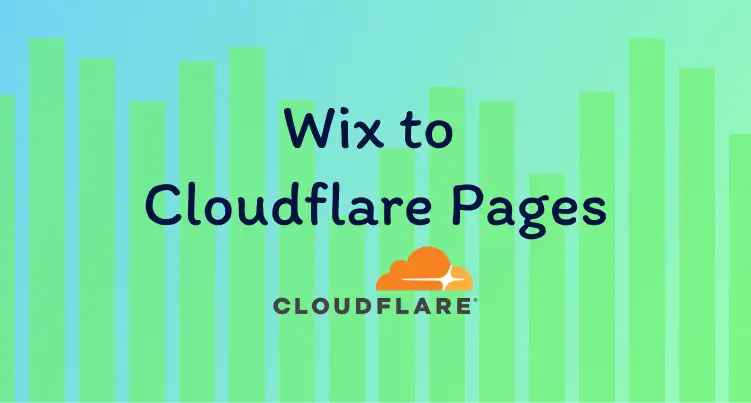
Migrating from Wix to a static site generator
23 April 2022
·I’m fed up with paying for Wix.
A couple of years ago I created a simple app to backtest copy trading. From this, I tried creating an affiliate site around copy trading and Etoro. It’s pretty simple stuff - just a couple of articles about copy trading. It was a complete fail but that’s not the point. For the site, I chose what I thought was the simplest solution at the time: Wix.
Wix is a decent website builder but like all website builders, it costs money. I think my yearly bill is £122. I could just delete the site but I might want to pick it back up one day. So I want to keep it up, just not pay for it :D
Over the past couple of weeks, I’ve been doing more research into the JAMStack and static site generators. The more I learn about them the more I can’t stomach paying for another year of Wix. Especially because it’s literally only hosting a couple of articles. With a static site generator and Cloudflare Pages, I could be hosting this thing for free.
Picking a setup and template
There’s a tone of static site generators to choose from. You can even use Next or Nuxt to generate static sites. For this project, I want the simplest solution so I chose Jekyll. As the original generator, it’s now the most popular and widely supported one out there
I’ve already mentioned Cloudflare Pages in the introduction. It’s a no-brainer choice for me to use them to host the site. I played around with them in my previous blog post where I did a bit of a comparison between Netlify and Cloudflare. I was very impressed with Cloudflare’s free plan, it’s really really good and perfect for hosting this project.
An advantage of picking Jekyll is that there are plenty of good free themes to choose from. I wat a simple blog-focused theme that is nice and clean. I found one appropriately called Affiliates which fits this description perfectly. You can find it here: https://jekyllthemes.io/theme/affiliates
Screenshot of the Affiliates theme
Cloning and editing the template to the theme of my site was straight forward. The template is well structured and documented with code comments.
Exporting articles and images from Wix
Getting your blog posts out of Wix is really annoying. They don’t provide an export feature which is just silly…
Jekyll has a collection of importers to convert sites like WordPress to a Jekyll static site. It doesn’t have one for Wix directly but it does have one for RSS feeds. Wix automatically publishes all blog posts to a feed hosted at yoursite.com/blog-feed.xml.
I tried the RSS importer but it didn’t work. First, it didn’t work with the latest version of Ruby. Then when I downgraded Ruby the importer didn’t import any of the content, just the titles. Looks like I need to write something myself…
That Gif is a bit overdramatic…. it wasn’t too bad.
I was able to whip something up in C# reasonably quickly. C# has good built-in methods for dealing with XML. So traversing the feed is not a problem. For the content I found two useful libraries: HtmlAgilityPack that can extract image links from HTML and Html2Markdown to convert the HTML blog posts to markdown.
The RSS of your Wix site contains each article in a list of item elements. Each of these items contains the article title, content and other metadata. This is the C# I used to read the feed, loop through the blog posts and pull out the meta data we need:
XmlReader xmlReader = XmlReader.Create(new Uri(BlogUrl, "/blog-feed.xml").ToString());
while (xmlReader.ReadToFollowing("item"))
{
xmlReader.ReadToFollowing("title");
var title = xmlReader.ReadElementContentAsString();
xmlReader.ReadToFollowing("pubDate");
var publishedDate = DateTime.Parse(xmlReader.ReadElementContentAsString());
// Get Featured image
var featImg = xmlReader.GetAttribute("url");
// ....
}
An important part was to download all the images from Wix. You don’t want your static site to still be referencing images hosted on their servers. Parsing the content of the article with HtmlAgilityPack lets us select all of the IMG elements and download the images. Then we replace the Wix URL in the article content with the Jekyll version:
// Extract and download post images
foreach (var element in doc.DocumentNode.Descendants("img"))
{
var imageUrl = element.Attributes["src"].Value;
imgCount++;
var img = await DownloadImage(DownloadLocation, imageUrl, imgCount.ToString());
element.Attributes["src"].Value = $"/assets/images/{img}";
}
Finally, we can convert the article HTML to Markdown and prepend the article metadata:
var markdown = converter.Convert(Encoding.ASCII.GetString(memStream.ToArray()));
markdown = "---\n" + markdown;
markdown = $"image: {featImg}" + "\n" + markdown;
markdown = "categories: [ Etoro, Copy Trading ]\n" + markdown;
markdown = "author: liam\n" + markdown;
markdown = $@"title: ""{title}""" + "\n" + markdown;
markdown = "layout: post\n" + markdown;
markdown = "---\n" + markdown;
File.WriteAllText(Path.Combine(DownloadLocation, $"markdown/{publishedDate.Year}-{publishedDate.Month}-{publishedDate.Day}-{title.Replace("?", "").Replace(":", "").Replace(" ", "-").ToLower()}.md"), markdown);
The full code is available on Github if you want to use it. You may have to customize it depending on your site theme.
https://github.com/Liam-Hunt/wix-to-markdown
Now we have the blog posts in markdown they can be copied into the Jekyll site.
Deployment
Deployment couldn’t be easier with Cloudflare pages. You just need to select your Github repo, set the framework, and give the site a name. Cloudflare will build and deploy the site in a couple of minutes.
Site comparison
Performance is important for all websites. Long loading times can make users close your website before they even read it. This is where static sites has an advantage. Hosted at the edge they have incredible speed. My current Wix site doesn’t feel slow but I will be interested to see how it compares.
At this point, we have the new static site deployed to a .pages.dev Cloudflare URL that we can use for testing. I’m going the same blog post on each site through the Web.dev testing tool.
Original Wix site:
I’m surprised how bad Wix scored for performance. 24 is a very low score. It shows the site would suck for users loading it over a slow mobile connection.
The biggest opportunity identified is “Reduce initial service response time”. This is the time it takes Wix’s server to respond to the request, something I have no control over to improve.
Static site version:
The static site is looking good! High scores across the board. Performance has dramatically improved with the simplified site served from Cloudflare’s edge network.
The biggest opportunities are now related to the images in the posts. This makes sense because I’ve just stuck the raw images into the articles. Optimizing images in Jekyll is something to learn and try out in the future. I think the current performance is satisfactory for now :P
Handling URL redirects
When migrating any site we need to be careful not to break any existing links. This can frustrate users that have bookmarked those links but more importantly, it can upset Google.
The difference between the two versions is small. When copying the posts from the Wix RSS feed the markdown files were named after the post title. Wix does the same thing. The only difference now is that Wix includes a /post/ before the title in the URL.
There are a few ways we could fix this. We could just structure our static site in the same way as Wix. So include a /post. for our posts. But I thought it would be cool though to use Cloudflare Pages redirects.
All we need to do is add a _redirects file to the site which is a list of all our redirects. In ours we just need one line:
/post/* /:splat 301
This will redirect all requests going to a URL starting with /post. The * and :splat means that anything following /post will get copied to the new URL.
For example:
https://www.spbuilder.io/post/etoro-who-to-copy
Will get redirected to
https://www.spbuilder.io/etoro-who-to-copy
The _redirects file can live in the root of the project then get copied to the Jekyll site output. To get it copied add these lines to the _config.yml:
include: - _redirects
Now any request going to a /post URL should get redirected.
Repointing DNS
To repoint the URL to the static site we need to transfer the DNS from Wix to Cloudflare. This process is very easy. There won’t be any downtime because when you add the domain to Cloudflare it will copy all the current DNS records. So any requests will still be sent to Wix until the redirect is complete.
Part of migrating the DNS is switching the domain’s name servers. You need to go to where you bought the domain and replace the name servers with Cloudflare’s.
Cloudflare gives you exact instructions when going through the process:
Migrating DNS to Cloudflare
It takes a while for registrars to process name server changes. Cloudflare will check the status periodically and notify you when it’s complete.
When the registrar updates and Cloudflare controls the DNS the domain can be added to the static site:
Adding a custom domain to Cloudflare pages
Cloudflare will repoint the URL and the static site will become the main site. woohoo!
And now I can cancel my Wix subscription :)
Canceling my wix subscription
So that’s £122 saved and the site is faster! Once again Cloudflare has shown how awesome they are. Incredible hosting and tools all for free.
Hope this post helped anyone else trying to escape Wix :P